How to develop Scalable Programs as a Developer By Gustavo Woltmann
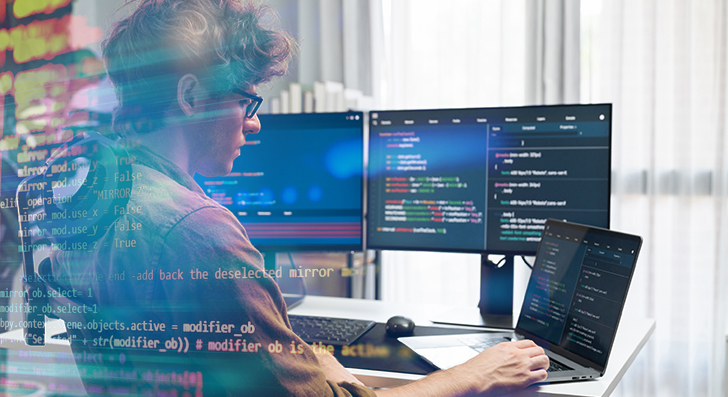
Scalability implies your software can take care of progress—much more users, additional knowledge, and even more visitors—without breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Right here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fall short every time they expand speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you have to Consider early regarding how your program will behave stressed.
Start by planning your architecture to be flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle a million consumers or merely 100? Pick the right kind—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t require them however.
Yet another crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than current conditions. Consider what would occur In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that assist scaling, like concept queues or function-driven techniques. These aid your app handle more requests without getting overloaded.
After you Establish with scalability in your mind, you're not just getting ready for achievement—you are decreasing long term headaches. A perfectly-prepared technique is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is usually a essential Portion of developing scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as result in failures as your application grows.
Start off by knowing your data. Could it be very structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are typically robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and facts.
In case your details is more versatile—like user action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, take into account your read and compose styles. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be dealing with a significant write load? Explore databases which will tackle higher publish throughput, or maybe event-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider forward. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your access patterns. And usually check database efficiency as you expand.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of difficulty afterwards.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, every small hold off provides up. Badly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated Alternative if an easy 1 works. Maintain your functions shorter, targeted, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes also long to operate or utilizes far too much memory.
Up coming, look at your database queries. These often sluggish things down a lot more than the code itself. Be sure each question only asks for the info you really have to have. Steer clear of Pick out *, which fetches every little thing, and in its place choose precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, Specially throughout big tables.
When you recognize a similar information currently being asked for again and again, use caching. Retailer the final results temporarily making use of instruments like Redis or Memcached so you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app much more productive.
Make sure to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash every time they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when required. These measures support your application keep easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and even more targeted traffic. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming site visitors throughout several servers. As opposed to 1 server performing all the do the job, the load balancer routes people to diverse servers determined by availability. This implies no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily get more info based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When buyers request the same information and facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it through the cache.
There are two prevalent varieties of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more economical.
Use caching for things that don’t transform frequently. And generally make sure your cache is up-to-date when details does modify.
Briefly, load balancing and caching are easy but strong applications. With each other, they assist your application deal with far more buyers, stay quickly, and Get better from problems. If you plan to increase, you would like equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app expand simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors improves, you can add more resources with just a few clicks or automatically utilizing auto-scaling. When traffic drops, you can scale down to save money.
These platforms also give services like managed databases, storage, load balancing, and stability applications. You may center on constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container deals your app and every thing it needs to operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your notebook to your cloud, with no surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You may update or scale elements independently, which is great for performance and dependability.
In short, employing cloud and container tools suggests you are able to scale rapid, deploy effortlessly, and Get well quickly when challenges happen. In order for you your application to expand without the need of limitations, start out using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not fixing.
Keep an eye on All the things
In the event you don’t keep an eye on your software, you won’t know when issues go Mistaken. Checking helps you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—check your app way too. Control just how long it requires for end users to load web pages, how frequently problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This will help you resolve concerns quick, often right before people even observe.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to serious problems.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct applications in position, you continue to be on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the ideal resources, you may Develop applications that mature smoothly with no breaking stressed. Begin modest, think huge, and Make smart.